Live Streaming Video Chat App without voice using cv2 module of Python
In this article, we will be creating a live Video Chat App using the OpenCV library and Socket Programming.
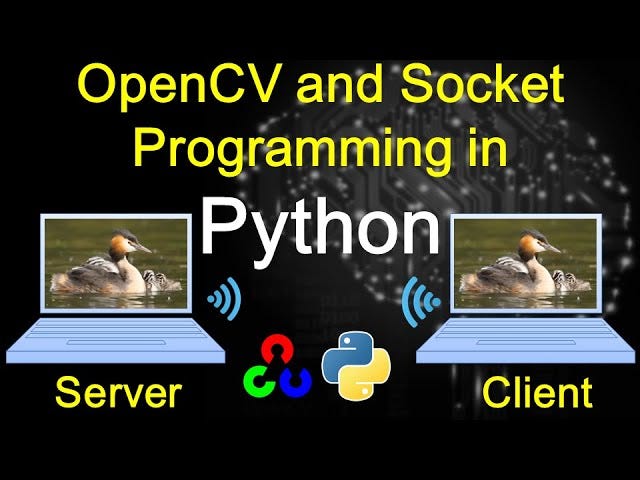
What is OpenCV library?
OpenCV (Open Source Computer Vision Library) is a library of programming functions mainly aimed at real-time computer vision.
What is Socket Programming?
Socket programming is a way of connecting two nodes on a network to communicate with each other. One socket(node) listens on a particular port at an IP, while the other socket reaches out to the other to form a connection. The server forms the listener socket while client reaches out to the server.

Let’s jump to the task :)
I m using windows cmd prompt for executing this task.
Prerequisite:
- Python and Necessary libraries (Numpy and OpenCV) should be installed.
For this, we will be creating code for the server-side and client-side.
SERVER SIDE CODE
import os
import cv2
cap=cv2.VideoCapture(1)
import numpy as np
import socket
# Create Socket
s=socket.socket(socket.AF_INET, socket.SOCK_STREAM)
ip="192.168.43.147"
port=8888
# Socket Binding
s.bind((ip,port))
s.listen(5)
# Listening and waiting for connection
conn,addr = s.accept()
while True:
data = conn.recv(90456)
# Decode the image
arry = np.fromstring(data, np.uint8)
photo = cv2.imdecode(arry, cv2.IMREAD_COLOR)
if type(photo) is type(None):
pass
else:
cv2.imshow("SERVER-SCREEN",photo)
if cv2.waitKey(10)==13:
break
stat,photo=cap.read()
# Encode image and send via network
photo_data = cv2.imencode('.jpg', photo)[1].tobytes()
conn.sendall(photo_data)
cv2.destroyAllWindows()
cap.release()
os.system("cls")
CLIENT SIDE CODE
import os
import cv2
cap=cv2.VideoCapture(0)
import numpy as np
import socket
# Client socket created
s=socket.socket(socket.AF_INET, socket.SOCK_STREAM)
ip="192.168.43.147"
port=8888
# Connection to server
s.connect((ip,port))
while True:
stat,photo=cap.read()
# Encode and send data via network
photo_data = cv2.imencode('.jpg', photo)[1].tobytes()
s.sendall(photo_data)
data = s.recv(90456)
# Decode the image
arry = np.fromstring(data, np.uint8)
photo = cv2.imdecode(arry, cv2.IMREAD_COLOR)
if type(photo) is type(None):
pass
else:
cv2.imshow("CLIENT-SCREEN",photo)
if cv2.waitKey(10)==13:
break
cv2.destroyAllWindows()
cap.release()
os.system("cls")
For getting a live video chat app in ur system just pull the code from the below-mentioned Github URL and replace the IP address with ur system’s IP address and run it in two different cmd prompt terminals.
GitHub_URL: https://github.com/nirali1219/MLOPS_Task_3
So here is our own Live Streaming Video Chat App.